Overview
Introduction
HireRight Connect™ is a RESTful API-based integration platform that makes it easy for clients and partners to build integrations using standard technologies. It exposes critical functions necessary to place and track background screening orders and I-9 orders providing a similar user experience and feature set to our exiting solutions. This API enables you to integrate HireRight background checks and/or I-9s with a client system; typically some form of ATS (Applicant Tracking System), but it can also be any other system.
The following high-level architecture diagram shows the flow between client code, Azure, and HireRight:
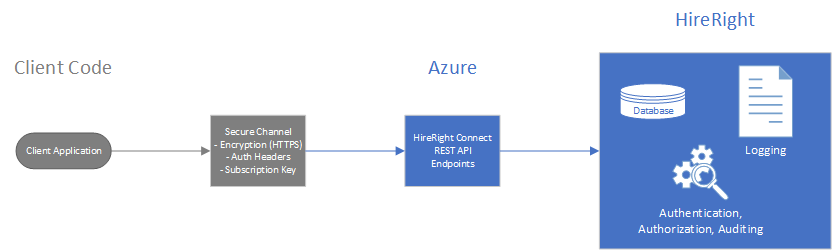
Client Code: Calls the HireRight Connect REST API endpoints on Azure over a secure channel
Azure: Exposes multiple HireRight Connect REST endpoints to the client application for consuming HireRight services
HireRight: Implements all available back-end services, including security, logging, and database access
Orders, Status, and Reports
The primary activities performed through the API are:
These links provide a quick overview for these activities and explain how to implement your own interfaces.
Common Operations
Detailed descriptions of individual operations can be found by browsing the resource pages.
Background & Drug Screening:
POST Orders: Creates order and returns the HireRight Order ID and the list of products being ordered. In the case of an error, a fallback response will be returned.
GET /orders/{id}/status: Returns the status summary for an order with the given Order ID. The status includes Order Status, Adjudication Status, and an array of Product Statuses.
GET /orders/{id}/report: Returns the report for an order with the given Order ID. Includes an option to get the report PDF in BASE64 format.
POST /orders/{id}/report-links/{type}: Returns a Single Sign On (SSO) link to a background screen report for an order with the given id. The type parameter indicates the type of report.
POST /web-links/{website}: Returns a Single Sign On (SSO) link for a given website. The website parameter indicates the relevant website.
GET /packages: Returns the list of available packages.
GET /products: Returns the list of available products.
GET /orders/{id}/documents: Returns all the relevant supporting documents for the order with the given Order ID.
POST /orders/search: Returns the list of orders matching the provided criteria.
GET /orders/{id}/adjudication-statuses: Returns all the relevant adjudication statuses for the order with the given Order ID.
PATCH /orders/{id}/adjudication-statuses: Update an adjudication status for the order with the given Order ID.
GET /orders/status-updates: Fetches all accumulated order status updates since the previous request. Note that once the statuses are sent back to the client, they are gone.
GET /accounts: Returns a list of company accounts.
POST /dhs/collection-sites: Returns a list of collection sites, along with the relevant information for each collection site.
GET /dhs/{id}/documents?type={type}: Returns DHS related documents according to the document type and an order id.
I-9s:
POST /I-9: Creates a new I-9 Form and returns the created Form ID.
GET /I-9/{id}/employee?email=<employeeEmail>: Returns a Single Sign-On (SSO) weblink that will redirect the employee to the Section 1 Worksheet. The 'email' parameter value is required and must match the employee's email address provided in the POST /I-9 operation.
GET /I-9/{id}/employer: Returns a Single Sign-On (SSO) weblink that will redirect the employer to either the Section 2 Worksheet or the Completed I-9 form, depending on the I-9 form’s status with HireRight.
GET /I-9/{id}/weblink: Returns a Single Sign-On (SSO) weblink that will redirect the employer to I-9 Form PDF.
GET /I-9/{id}/form: Returns a base64 encoded pdf file of the I-9 form.
GET /I-9/{id}/status: Returns the status summary for an I-9 form with the given Form ID. The status includes I-9 status and E-Verify status if applicable.
GET /I-9/{id}/documents: Returns all supporting documentation for an I-9 form with the given Form ID.
General Info
Request Header
Clients must provide the following information in each request header to identify themselves, the user, and the API version used. All of these values are provided by HireRight.
hr-domain-id: This is the unique API integration domain identifier.
hr-company-login: This is a unique identifier that identifies the client’s company.
hr-user-ref-id: This is a unique identifier of the user in the client’s system.
hr-api-subscription-key: This is the specific integration key that provides access to the API.
hr-api-version: This is the specific version of the API to be used.
User Ref Id
To ensure that requests are granted proper permissions, it is imperative that the proper User Ref Id is passed to the service. This value is a unique ID assigned to every user and is typically set to the user's verified email address or username. This value must be passed to HireRight in each request header as hr-user-ref-id. HireRight Connect then uses that value to uniquely identify the user in your account, which ultimately determines the specific privileges that are available to the request.
For example:
Sample User 1:
Jane Doe is the HR Manager for “A-One Products and Services,” and is responsible for all hiring.
Jane’s verified email address in your system is Jane.Doe@A-One.com, which will be used as Jane's unique identifier for HireRight Connect requests.
As HR Manager, Jane must be able to order background checks from HireRight and must also be able to view background reports of all applicants.
Sample User 2:
Michael Adams is a temp HR Analyst contracted to help out because of a busy hiring season.
Michael’s verified unique email address in your system is Michael.Adams@A-One.com, which will be used as Michael's unique identifier for HireRight Connect requests.
As a temp analyst, Michael should also be able to order background checks from HireRight but should not be able to view background reports.
To support this scenario, HireRight provides a single company-wide account for A-One.com. Within this account, profiles for Jane Doe and Michael Adams are provided as Jane.Doe@A-One.com and Michael.Adams@A-One.com, respectively. Michael’s profile will be only granted the privilege to Create Orders. Jane will be able to Create Orders and View Reports. Therefore, it is critical to pass this value via hr-user-ref-id in each request header based on who is currently logged in and ensure that no other value is ever used during the lifetime of the session. In this scenario, doing so ensures that Michael cannot view reports, while Jane can.
Rate Limits
In order to provide a high-quality of service for all customers and partners that utilize an integration with HireRight, the following rate limits are in place:
100 requests per minute:
POST /orders
POST /I-9
50 requests per minute:
GET /orders/{id}/report
GET /orders/{id}/status
GET /I-9{id}/status
POST /orders/{id}/report-links/{type}
If the rate limit is reached HireRight will respond with a HTTP status code 429 "Too Many Requests".
HireRight reserves the right to adjust the rate limit for any given endpoint in the future as needed.
Error Handling
The API uses common error codes and when relevant also includes additional information in the response body.
The general error response body is as described below:
{
"statusCode" : 400,
"message" : "string",
"exchangeId" : "string",
"traceId" : "string",
"transactionId" : "string"
}
It is recommended to handle errors appropriately by displaying relevant error messages to the user:
When getting the general error, the response message will include the error description which is recommended to be displayed to the user
In addition, when a transaction id is provided, it is recommended to display it to the user since it can assist troubleshooting on the HireRight side when applicable
Please note that some errors, such as fields validation and duplicates detection errors of the order creation endpoint, may have a specific format. You can find those on the relevant endpoint page.
Common Errors
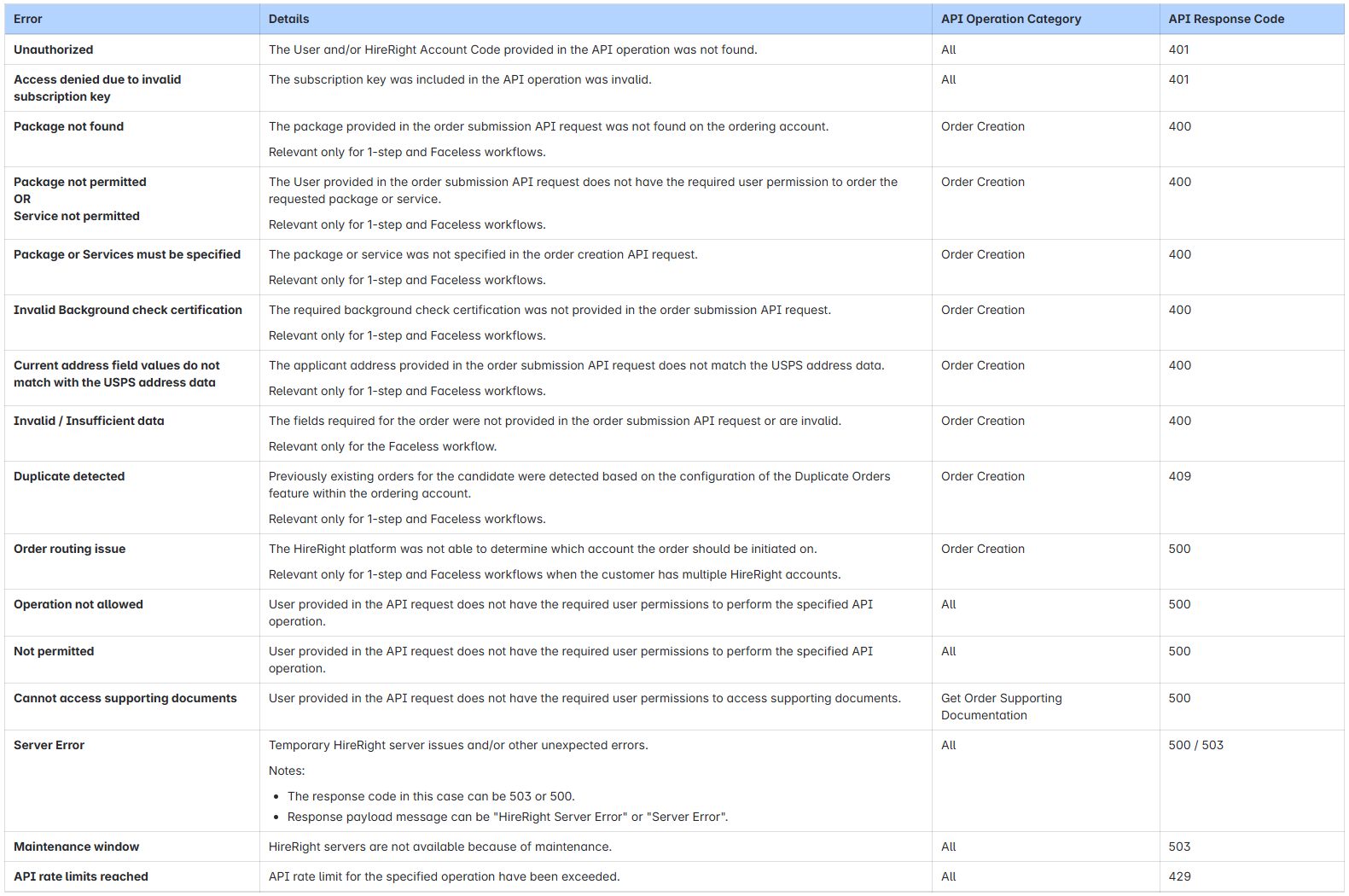